Re: database type script [message #231150 is a reply to message #231091] |
Sun, 19 November 2006 08:59  |
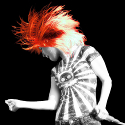 |
danpaul88
Messages: 5795 Registered: June 2004 Location: England
Karma:
|
General (5 Stars) |
|
|
Ah, well in that case your in luck, PHP's arrays are MUCH easier to work with than many other languages, since you don't need to specify a fixed size for them.
Reading from a file every time you want to do something is a waste of CPU time, its better to store it in an array, and put it into a file for permanent storage when the script shuts down, or every x minutes as a backup.
First thing you need is a system for incrementing the name. I will leave it to you to setup when that is done, but this is the code to do it, assuming $playerName contains the players username, and the array is called $playerCountArray. Again, all code is case-insensitive.
if ( array_key_exists( strtolower($playerName), $playerCountArray )
{
$playerCountArray[strtolower($playerName)]++;
}
else
{
$playerCountArray[strtolower($playerName)] = 1;
}
Basically this looks through the array to see if they are already in there. If they are it simply increments their value, otherwise it creates them and sets their value to one. In PHP the syntax for creating an array key and modifying it is the same.
Next you need to be able to dump to a file when the bot closes, or however often you want to save the array. This is a quick file dump method for the array. We set the variable to "" first because PHP does not discard variables when it's finished with them, so we need to ensure it is empty before we start. The .= operator is the append operator.
$fileContents = "";
foreach ( $playerCountArray as $player => $count )
{
$fileContents .= $player . " = " . $count . "\n";
}
$fileHandle = fopen ( "logfile.txt", "w" );
fwrite ( $fileHandle, $fileContents );
fclose ( $fileHandle );
The resulting file will be called logfile.txt and will look like this;
player1 = 5
player2 = 8
player3 = 3
Finally, you need a way to read the values back from the file when the bot is restarted.
$fileContentsArray = explode ( "\n", file_get_contents ( "logfile.txt" ) );
foreach ( $fileContentsArray as $line )
{
list( $player, $count ) = explode ( " = ", $line );
$playerCountArray[$player] = $count;
}
Hope that helps you out.
[Updated on: Sun, 19 November 2006 09:00] Report message to a moderator
|
|
|