|
|
|
|
|
|
Re: Veteran Plugin. [message #331345 is a reply to message #300286] |
Tue, 20 May 2008 17:59   |
slavik262
Messages: 79 Registered: February 2007
Karma: 0
|
Recruit |
|
|
www.learncpp.com is the path I took, along with C++ for dummies. However, I already had some experience in C. I've done no Renegade scripting, but from the looks of it, scripting is very template driven and object-oriented.
|
|
|
|
Re: Veteran Plugin. [message #331766 is a reply to message #300286] |
Sat, 24 May 2008 07:44   |
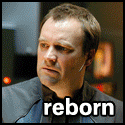 |
reborn
Messages: 3231 Registered: September 2004 Location: uk - london
Karma: 0
|
General (3 Stars) |
|
|
I decided to upgrade the veteran system that I posted here to use in cnc_reborn. I changed it so that it rewards players for healing/repairing and disarming.
I also added some little notices for players too, these notices will only be displayed for people using the upgraded client scripts.dll, but for cnc_reborn that's fine.
Here is the changes I made.
struct RepPlayers {
std::string RepPlayerName;
int RepPoints;
};
std::vector<RepPlayers> RepInfo;
void RepUpdate(int ID, int number) {
if (!RepInfo.empty()) {
for (int i = 0; i < RepInfo.size(); i++) {
if (RepInfo[i].RepPlayerName == Get_Player_Name_By_ID(ID)) {
int cvp, now;
cvp = RepInfo[i].RepPoints;
now = number + cvp;
RepInfo[i].RepPoints = now;
if (cvp < 200 && now >= 200) {
VetUpdate(ID, 1, Commands->Get_Points(Get_GameObj(ID)));
Display_Int_Player(Get_GameObj(ID),1,"Bonus repairing veteran points gained: 1");
RepInfo[i].RepPoints = 0;
now = 0;
cvp = 0;
}
break;
}
}
}
}
int RepCheckPoints(int ID) {
if (!RepInfo.empty()) {
for (int i = 0; i < RepInfo.size(); i++) {
if (RepInfo[i].RepPlayerName == Get_Player_Name_By_ID(ID)) {
int Points;
Points = RepInfo[i].RepPoints;
return Points;
}
}
}
return 0;
}
bool RepCheck(int ID) {
if (!RepInfo.empty()) {
for (int i = 0; i < RepInfo.size(); i++) {
if (RepInfo[i].RepPlayerName == Get_Player_Name_By_ID(ID)) {
return true;
}
}
}
return false;
}
void RepAddPlayer(int ID) {
if (RepCheck(ID) == false) {
RepPlayers temp;
temp.RepPlayerName = Get_Player_Name_By_ID(ID);
temp.RepPoints = 0;
RepInfo.push_back(temp);
}
}
void RepClearPlayers() {
RepInfo.erase(RepInfo.begin(), RepInfo.end());
int Players = The_Game()->MaxPlayers;
for (int i = 1; i <= Players; i++) {
if (Get_GameObj(i)) {
RepAddPlayer(i);
}
}
}
Then on the ::Damaged events for the vehicles, players and buildings I did the following:
void MDB_SSGM_Vehicle::Damaged(GameObject *obj, GameObject *damager, float damage) {
if(damage < 0){
if (Commands->Is_A_Star(damager) && Commands->Get_Player_Type(damager) == Commands->Get_Player_Type(obj)){
RepUpdate(Get_Player_ID(damager), ((damage - damage) - damage)/2);
}
}
if (damage > 0.0f) {
LastDamage = damage;
}
}
void MDB_SSGM_Player::Damaged(GameObject *obj, GameObject *damager, float damage) {
if(damage < 0){
if (Commands->Is_A_Star(damager) && Commands->Get_Player_Type(damager) == Commands->Get_Player_Type(obj)){
RepUpdate(Get_Player_ID(damager), ((damage - damage) - damage));
}
}
if ((IsSecondWind == true) && (Commands->Get_Health(obj) <= 25) && (Commands->Get_Health(obj) > 0)) {
IsSecondWind = false;
Commands->Set_Health(obj,Commands->Get_Max_Health(obj));
Commands->Set_Shield_Strength(obj,Commands->Get_Max_Shield_Strength(obj));
}
}
void MDB_SSGM_Building::Damaged(GameObject *obj, GameObject *damager, float damage) {
if(damage < 0){
if (Commands->Is_A_Star(damager) && Commands->Get_Player_Type(damager) == Get_Object_Type(obj)){
RepUpdate(Get_Player_ID(damager), ((damage - damage) - damage));
}
}
void MDB_SSGM_Beacon::Killed(GameObject *obj, GameObject *shooter) {
VetUpdate(Get_Player_ID(shooter), 5, Commands->Get_Points(shooter));
Display_Int_Player(shooter,5,"bonus veteran points gained: 5");
if (IsDisarmed == false) {
IsDisarmed = true;
FDSMessage(StrFormat("%ls disarmed a %s",Get_Wide_Player_Name(shooter),Translate_Preset(obj).c_str()),"_BEACON");
}
}
void MDB_SSGM_C4::Killed(GameObject *obj, GameObject *shooter) {
VetUpdate(Get_Player_ID(shooter), 1, Commands->Get_Points(shooter));
Display_Int_Player(shooter,1,"Bonus veteran points gained: 1");
if (Settings->LogC4) {
FDSMessage(StrFormat("%ls %s has been disarmed by %ls (Owner: %ls - Attached to: %s)",Get_Wide_Team_Name(Get_Object_Type(obj)),Translate_Preset(obj).c_str(),Get_Wide_Player_Name(shooter),Get_Wide_Player_Name(Get_C4_Planter(obj)),Translate_Preset(Get_C4_Attached(obj)).c_str()),"_C4");
}
WasDisarmed = true;
}
I added the script to players on the join hook, the same as the veteran thing, here:
void Player_Join_Hook(int i,const char *Nick) {
VetAddPlayer(i);
RepAddPlayer(i);
And I clear the info on the game over event here:
void GameOver() {
VetClearPlayers();
RepClearPlayers();
char ObjectsType[10],ObjectsType2[10],ObjectsFile[20];
I also added those little messages on the normal veteran points gained too, here:
void MDB_SSGM_Vehicle::Killed(GameObject *obj, GameObject *shooter) {
if (Commands->Is_A_Star(shooter)){
VetUpdate(Get_Player_ID(shooter), GetPoints(Commands->Get_Preset_Name(obj)), Commands->Get_Points(shooter));
Display_Int_Player(shooter,GetPoints(Commands->Get_Preset_Name(obj)),"Veteran points gained: %d"),GetPoints(Commands->Get_Preset_Name(obj));
void MDB_SSGM_Player::Killed(GameObject *obj, GameObject *shooter) {
if (Commands->Is_A_Star(shooter) && Commands->Get_Player_Type(shooter) != Commands->Get_Player_Type(obj))
{
VetUpdate(Get_Player_ID(shooter), GetPoints(Commands->Get_Preset_Name(obj)), Commands->Get_Points(shooter));
Display_Int_Player(shooter,GetPoints(Commands->Get_Preset_Name(obj)),"Veteran points gained: %d"),GetPoints(Commands->Get_Preset_Name(obj));
void MDB_SSGM_Building::Killed(GameObject *obj, GameObject *shooter) {
if (Commands->Is_A_Star(shooter)){
VetUpdate(Get_Player_ID(shooter), 25, Commands->Get_Points(shooter));
Display_Int_Player(shooter,25,"Veteran points gained: 25");
}
Hope someone finds a use for it.. I may even make it a plug-in if I have time in the next few days, maybe...
Also, my maths seems to be fading away from me...
There must be a better way to turn a negative number into a positive number, rather then "(damage - damage) - damage)".
|
|
|
|
Re: Veteran Plugin. [message #331770 is a reply to message #331766] |
Sat, 24 May 2008 08:36   |
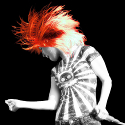 |
danpaul88
Messages: 5795 Registered: June 2004 Location: England
Karma: 0
|
General (5 Stars) |
|
|
reborn wrote on Sat, 24 May 2008 15:44 |
Also, my maths seems to be fading away from me...
There must be a better way to turn a negative number into a positive number, rather then "(damage - damage) - damage)".
|
#include <cstdlib>
abs(number);
or, for floating point numbers
#include <cmath>
fabs(number);
Or, if you already know for sure the number is negative;
int number = -number;
[Updated on: Sat, 24 May 2008 08:37] Report message to a moderator
|
|
|
|
Re: Veteran Plugin. [message #331923 is a reply to message #300794] |
Sun, 25 May 2008 15:37   |
lonleyppl
Messages: 4 Registered: May 2008
Karma: 0
|
Recruit |
|
|
not many people can make fun of your code reborn
it seems to me like you have coded half the modded servers ive played
one example
hunt the player (mvrtech)
awesome idea that no one else has had
|
|
|
|
|
|
Re: Veteran Plugin. [message #332962 is a reply to message #300286] |
Sun, 01 June 2008 17:36   |
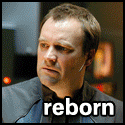 |
reborn
Messages: 3231 Registered: September 2004 Location: uk - london
Karma: 0
|
General (3 Stars) |
|
|
I'll be releasing the code that runs on the official server and the compiled scripts.dll, which is based on SSGM. I don't understand why you think I wouldn't :-/
This is getting rather off-topic though.
|
|
|
|
Re: Veteran Plugin. [message #333067 is a reply to message #300286] |
Mon, 02 June 2008 23:37   |
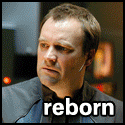 |
reborn
Messages: 3231 Registered: September 2004 Location: uk - london
Karma: 0
|
General (3 Stars) |
|
|
Yeah, that's possible. You could make the reward directly proportionate to the veteran level itself or the veteran points they have.
I'll throw something together...
|
|
|
|
Re: Veteran Plugin. [message #335007 is a reply to message #330148] |
Thu, 12 June 2008 19:16   |
HeavyX101- Left
Messages: 633 Registered: April 2008 Location: WindowsJail=ZipFolder
Karma: 0
|
Colonel |
|
|
EA-DamageEverything wrote on Sun, 11 May 2008 22:04 | error C2653: 'reb_vet_System' : is not a class or namespace name c:\ExScripts\SSGM Source\gmscripts.cpp 215
= paste the whole script into the gmmain.cpp and the class into gmmain.h, this could/should help. I do it this way and everything works.
--------
error C2601: 'GetPoints' : local function definitions are illegal c:\ExScripts\SSGM Source\gmscripts.cpp
= Get_Points would be a valid scripts command.
Over all, the whole code should be placed in one file. You cannot split Reborns' main code because the gmmain.cpp has zero access to the gmscripts.cpp if I am interpreting the include-Syntax right. In the other way, the gmscripts can read gmmain, gmcrate etc.
I probably fail with this post, but I do think it goes in the right direction.
|
Ok, ive copied and pasted the whole script into the gmmain.cpp and class into gmmain.h but i got 45 errors/warnings
Here they are
HERE!!!! ------ Build started: Project: SSGM, Configuration: Debug Win32 ------
Compiling...
gmmain.cpp
.\gmmain.cpp(1466) : error C2220: warning treated as error - no 'object' file generated
.\gmmain.cpp(1466) : warning C4018: '<' : signed/unsigned mismatch
.\gmmain.cpp(1525) : warning C4018: '<' : signed/unsigned mismatch
.\gmmain.cpp(1538) : warning C4018: '<' : signed/unsigned mismatch
.\gmmain.cpp(1735) : warning C4805: '==' : unsafe mix of type ''unknown-type'' and type 'bool' in operation
.\gmmain.cpp(1735) : error C3861: 'IsLowClassVehicle': identifier not found
.\gmmain.cpp(1746) : warning C4805: '==' : unsafe mix of type ''unknown-type'' and type 'bool' in operation
.\gmmain.cpp(1746) : error C3861: 'IsLowClassVehicle': identifier not found
.\gmmain.cpp(1759) : warning C4805: '==' : unsafe mix of type ''unknown-type'' and type 'bool' in operation
.\gmmain.cpp(1759) : error C3861: 'IsLowClassVehicle': identifier not found
.\gmmain.cpp(1772) : warning C4805: '==' : unsafe mix of type ''unknown-type'' and type 'bool' in operation
.\gmmain.cpp(1772) : error C3861: 'IsLowClassVehicle': identifier not found
.\gmmain.cpp(1777) : warning C4805: '==' : unsafe mix of type ''unknown-type'' and type 'bool' in operation
.\gmmain.cpp(1777) : error C3861: 'IsLowClassVehicle': identifier not found
.\gmmain.cpp(1782) : warning C4805: '==' : unsafe mix of type ''unknown-type'' and type 'bool' in operation
.\gmmain.cpp(1782) : error C3861: 'IsLowClassVehicle': identifier not found
.\gmmain.cpp(1842) : error C2084: function 'void Player_Join_Hook(int,const char *)' already has a body
.\gmmain.cpp(689) : see previous definition of 'Player_Join_Hook'
.\gmmain.cpp(1860) : error C2084: function 'void GameOver(void)' already has a body
.\gmmain.cpp(839) : see previous definition of 'GameOver'
.\gmmain.cpp(1899) : error C2653: 'vet_MDB_SSGM_Building' : is not a class or namespace name
.\gmmain.cpp(1899) : error C2601: 'Killed' : local function definitions are illegal
.\gmmain.cpp(1860): this line contains a '{' which has not yet been matched
.\gmmain.cpp(1926) : error C2065: 'vet_MDB_SSGM_Building' : undeclared identifier
.\gmmain.cpp(1926) : error C2514: 'ScriptRegistrant' : class has no constructors
c:\westwood\renegadefds\server\scripts.h(480) : see declaration of 'ScriptRegistrant'
.\gmmain.cpp(1929) : error C2063: 'Killed' : not a function
.\gmmain.cpp(1929) : error C2601: 'vet_MDB_SSGM_Building::Killed' : local function definitions are illegal
.\gmmain.cpp(1860): this line contains a '{' which has not yet been matched
.\gmmain.cpp(1930) : error C2065: 'shooter' : undeclared identifier
.\gmmain.cpp(1939) : error C2065: 'obj' : undeclared identifier
.\gmmain.cpp(1939) : error C2228: left of '.c_str' must have class/struct/union
.\gmmain.cpp(1942) : error C2228: left of '.c_str' must have class/struct/union
.\gmmain.cpp(1942) : error C2228: left of '.c_str' must have class/struct/union
.\gmmain.cpp(1948) : error C2065: 'IsAlive' : undeclared identifier
.\gmmain.cpp(1951) : error C2227: left of '->Destroy_Base' must point to class/struct/union/generic type
.\gmmain.cpp(1957) : error C2514: 'ScriptRegistrant' : class has no constructors
c:\westwood\renegadefds\server\scripts.h(480) : see declaration of 'ScriptRegistrant'
.\gmmain.cpp(1959) : error C2653: 'vet_MDB_SSGM_Player' : is not a class or namespace name
.\gmmain.cpp(1963) : error C3861: 'GetPoints': identifier not found
.\gmmain.cpp(1965) : error C2065: 'WasKilled' : undeclared identifier
.\gmmain.cpp(2023) : error C2065: 'vet_MDB_SSGM_Player' : undeclared identifier
.\gmmain.cpp(2023) : error C2514: 'ScriptRegistrant' : class has no constructors
c:\westwood\renegadefds\server\scripts.h(480) : see declaration of 'ScriptRegistrant'
.\gmmain.cpp(2025) : error C2653: 'vet_MDB_SSGM_Vehicle' : is not a class or namespace name
.\gmmain.cpp(2025) : error C2084: function 'void Killed(GameObject *,GameObject *)' already has a body
.\gmmain.cpp(1959) : see previous definition of 'Killed'
.\gmmain.cpp(2028) : error C3861: 'GetPoints': identifier not found
.\gmmain.cpp(2040) : error C2065: 'LastDamage' : undeclared identifier
.\gmmain.cpp(2180) : error C2065: 'vet_MDB_SSGM_Vehicle' : undeclared identifier
.\gmmain.cpp(2180) : error C2514: 'ScriptRegistrant' : class has no constructors
c:\westwood\renegadefds\server\scripts.h(480) : see declaration of 'ScriptRegistrant'
.\gmmain.cpp(2181) : fatal error C1075: end of file found before the left brace '{' at '.\gmmain.cpp(1860)' was matched
Creating browse information file...
Microsoft Browse Information Maintenance Utility Version 8.00.50727
Copyright (C) Microsoft Corporation. All rights reserved.
BSCMAKE: error BK1506 : cannot open file '.\tmp\scripts\debug\gmmain.sbr': No such file or directory
Build log was saved at "file://c:\Westwood\RenegadeFDS\Server\tmp\scripts\debug\BuildLog.htm"
SSGM - 36 error(s), 9 warning(s)
========== Build: 0 succeeded, 1 failed, 0 up-to-date, 0 skipped ==========
This account is no longer being active.
|
|
|
Re: Veteran Plugin. [message #335009 is a reply to message #335007] |
Thu, 12 June 2008 19:20   |
nopol10
Messages: 1043 Registered: February 2005 Location: Singapore
Karma: 0
|
General (1 Star) |
|
|
I think it would be good if you knew and understood the code rather than just copy and paste everything reborn puts up here.
You should check where you pasted the code, because you may have stuffed them into another method/function instead of in a blank space.
nopol10=Nopol=nopol(GSA)
|
|
|
Re: Veteran Plugin. [message #335010 is a reply to message #335009] |
Thu, 12 June 2008 19:35   |
HeavyX101- Left
Messages: 633 Registered: April 2008 Location: WindowsJail=ZipFolder
Karma: 0
|
Colonel |
|
|
I know where every thing goes. Most of the other commands are working. But when i tried doing this one, i had alot of errors. So i just read most of the replies and found this one and said that it might work. But it seems not to.
This account is no longer being active.
|
|
|
Re: Veteran Plugin. [message #335053 is a reply to message #300286] |
Fri, 13 June 2008 03:21   |
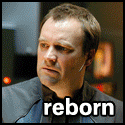 |
reborn
Messages: 3231 Registered: September 2004 Location: uk - london
Karma: 0
|
General (3 Stars) |
|
|
You're missing some functions, and your compiler is complaining about some warnings that in this instance do not really matter.
You're also missing at least one global variable, and I'm pretty sure you've either renamed some mdb scripts, or you've accidently missed a semi-colon or something.
|
|
|
|